Guide: How to use the AliExpress Affiliate API with Nodejs
Having trouble with making API requests to the AliExpress Affiliate API? In this blogpost I explain how to make API calls to the AliExpress API [including code samples!]
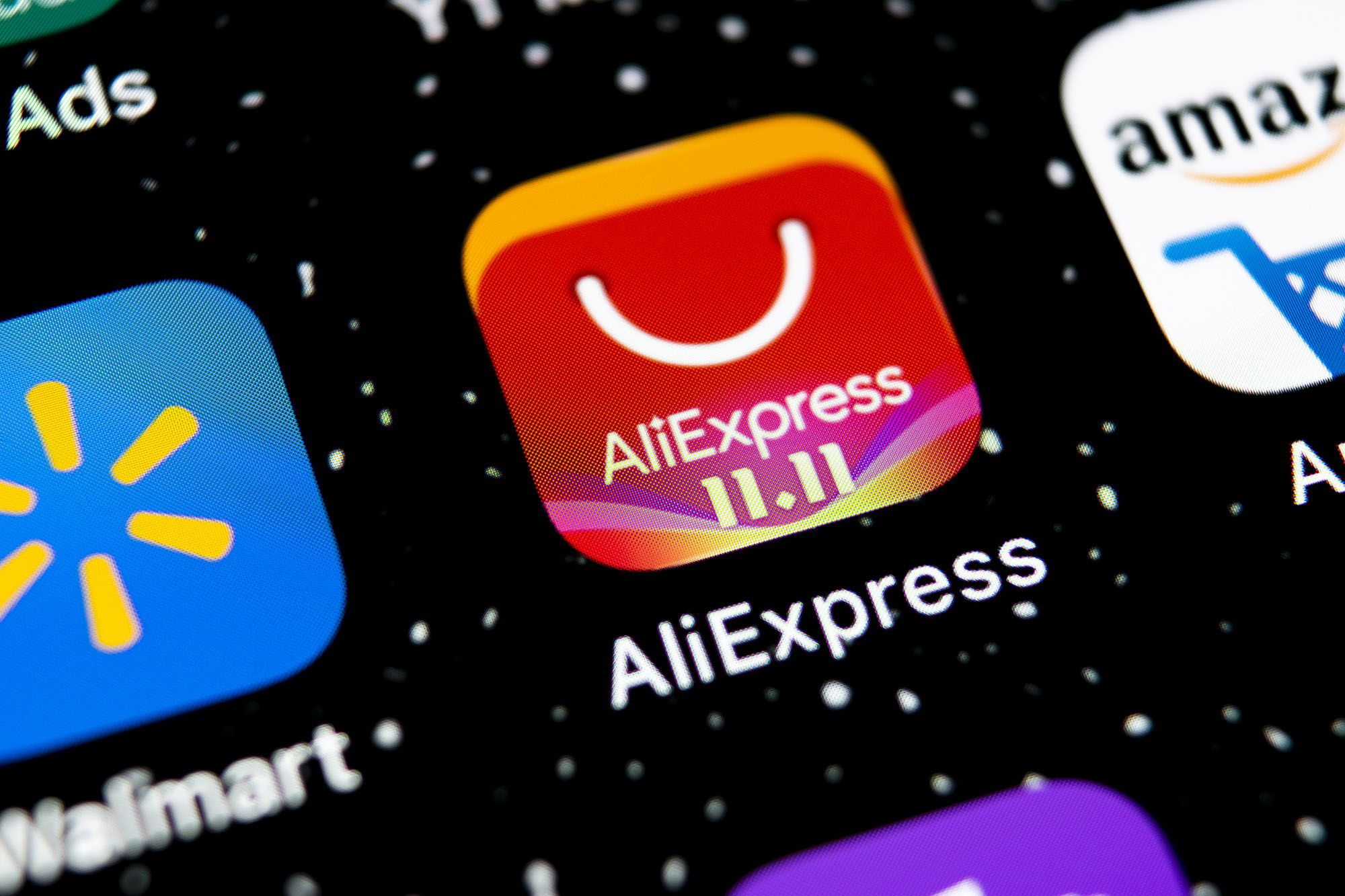
For my Aliexpress price tracker, AliWatcher, I wanted to implement the AliExpress API to not be solely dependant on datafeeds for the product data. With the API I can search product, fetch product information, get product recommendations, and more. Good stuff!
However, it took me some figuring out to get things working. The docs are mostly in Chinese (but readable/understandable with Google Translate), the signing of the API call was not 100% clear to me and the SDK was download only (no official NPM package).
So let's see how I managed to get it working, for example to get recommendations (as you can see in the bottom of any product on AliWatcher).
If you're having trouble to manage to get it working but you already made an account, you can just copy this code. Below the code I explain how to make a developer account, and go through the code line by line.
Code Sample
With this code sample you can fetch product detail of a product on AliExpress by ID. You can find the ID of a product in the URL, e.g https://www.aliexpress.com/item/4000669887458.html
I put the code in this blogpost as a Github gist here: How to make an API request to the AliExpress Affiliate API
If you have any questions about the code, feel free to comment on the gist!
import crypto from "crypto";
import dayjs from "dayjs";
import utc from "dayjs/plugin/utc";
import timezone from "dayjs/plugin/timezone";
dayjs.extend(utc);
dayjs.extend(timezone);
const API_URL = "http://gw.api.taobao.com/router/rest";
const API_SECRET = "FIND THIS IN THE AE CONSOLE";
const API_KEY = "FIND THIS IN THE AE CONSOLE";
const hash = (method, s, format) => {
const sum = crypto.createHash(method);
const isBuffer = Buffer.isBuffer(s);
if (!isBuffer && typeof s === "object") {
s = JSON.stringify(sortObject(s));
}
sum.update(s, "utf8");
return sum.digest(format || "hex");
};
const sortObject = (obj) => {
return Object.keys(obj)
.sort()
.reduce(function (result, key) {
result[key] = obj[key];
return result;
}, {});
};
const signRequest = (parameters) => {
const sortedParams = sortObject(parameters);
const sortedString = Object.keys(sortedParams).reduce((acc, objKey) => {
return `${acc}${objKey}${sortedParams[objKey]}`;
}, "");
const bookstandString = `${API_SECRET}${sortedString}${API_SECRET}`;
const signedString = hash("md5", bookstandString, "hex");
return signedString.toUpperCase();
};
export const getProductById = async (productId: string) => {
const timestamp = dayjs().tz("Asia/Shanghai").format("YYYY-MM-DD HH:mm:ss");
const payload = {
method: "aliexpress.affiliate.productdetail.get",
app_key: API_KEY,
sign_method: "md5",
timestamp,
format: "json",
v: "2.0",
product_ids: productId,
target_currency: "USD",
target_language: "EN",
};
const sign = signRequest(payload);
const allParams = {
...payload,
sign,
};
const res = await fetch(API_URL, {
method: "POST",
headers: {
"Content-Type": "application/x-www-form-urlencoded;charset=utf-8",
},
body: new URLSearchParams(allParams),
});
return await res.json()
}
Making an AliExpress Affiliate App
When you've created a developer account on AliExpress' developer platform you can go to the console. Remember, Google Translate is your friend if you don't speak any Chinese.
If you are redirected through Taobao.com, that's completely fine. I found that AliExpress and Taobao are really interwoven. Example of this in the code snippet above: to fetch product details from AliExpress we have to send an api request to the Taobao API.
When you're in the console you can create an app. The first thing required is to select the app type. For this we want the "Affiliates API".
When you created the app and click on it, you'll see the App Key and the App Secret. Those two you need to make calls to the AliExpress API.
To get access to the hot products query and the smart match api, you will need to obtain extra API permissions. The Standard API for Publishers is "gained" by default.

Making your first API call
The main docs for the AliExpress affiliate API can be found here.
These are (in my opinion) the most handy API endpoints:
API method | Action |
---|---|
aliexpress.affiliate.product.query | Search for a product by keyword, price, delivery days and country availability |
aliexpress.affiliate.hotproduct.query | Get the top selling products |
aliexpress.affiliate.productdetail.get | Get a product detail by product ID |
aliexpress.affiliate.product.smartmatch | Recommends product by either device_id (adid or idfa) or product_id (like a recommendation engine) |
Signing your API
const signRequest = (parameters) => {
const sortedParams = sortObject(parameters);
const sortedString = Object.keys(sortedParams).reduce((acc, objKey) => {
return `${acc}${objKey}${sortedParams[objKey]}`;
}, "");
const bookstandString = `${API_SECRET}${sortedString}${API_SECRET}`;
const signedString = hash("md5", bookstandString, "hex");
return signedString.toUpperCase();
};
The hardest part of the API was signing the API request. Per the docs here, this is how you sign a request:
- Check which parameters you need. The ones required are: method, app_key, timestamp (in the right timezone), v, sign_method and sign.
- Sort all the request parameters, minus the "sign" key.
- Then make a long string with all those parameters concatenated, just keyvaluekeyvaluekeyvalue
- As we use the
md5
algorithm to encode it, we create a new string where our previously created longstring of parameters is between our secret key, like two bookstands: secret_keylongstringsecret_key - Then hash the last string with the
md5
algorithm, tohex
. - This is now the value we send with our params as the
sign
key in our request - Now we send a
POST
request to the API endpoint (alwaysPOST
, even if the method looks like aGET
, e.g.aliexpress.affiliate.productdetail.get
. - Make sure the
Content-Type
in the headers equals toapplication/x-www-form-urlencoded;charset=utf-8
. - With
fetch
you can set the body asnew URLSearchParams(ourParams)
. If you use something likeaxios
, I assume it's the same but it's better to check the docs to make sure.
Remarks
I use dayjs
to create the correct timestamp with the right Timezone. However, you can use any dateformatting library (such as Momentjs) you are comfortable with. Just make sure the timestamp is in the format YYYY-MM-DD HH:mm:ss
.
I implemented the md5
hash method by myself, as I didn't get the crypto-js
hash method to work. If you're more familiar with crypto-js
than I am, feel free to comment how to do that on the github gist I created.
This is how I make API requests with my AliExpress Price Tracker called AliWatcher. With the API you can fetch product info, category information, top selling products, product recommendations and more.
I hope this guide helped you to make API requests.
Keep on hacking!
Jelle